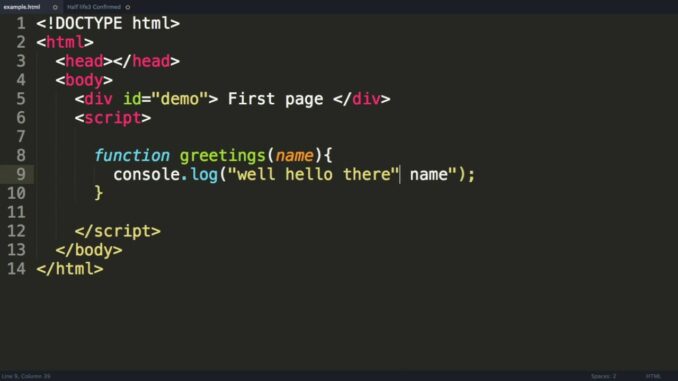
Functions are a fundamental concept in JavaScript and many other programming languages. They allow you to encapsulate code into reusable blocks, which helps in making your code more organized, maintainable, and efficient. Understanding how to create and use functions is crucial for writing effective JavaScript code.
In this comprehensive guide, we will explore everything you need to know about functions in JavaScript, including their creation, invocation, scope, and advanced concepts. We’ll cover the following topics:
- Introduction to Functions
- Creating Functions
- Function Declarations
- Function Expressions
- Arrow Functions
- The
Function
Constructor
- Invoking Functions
- Calling Functions
- Function Parameters
- Return Values
- Function Scope and Closures
- Local vs. Global Scope
- Closures
- Advanced Function Concepts
- Higher-Order Functions
- Callback Functions
- Function Currying
- IIFE (Immediately Invoked Function Expressions)
- Best Practices for Functions
- Common Pitfalls and Debugging Tips
- Conclusion
Introduction to Functions
Functions are blocks of code designed to perform a specific task. They can be executed when needed, and they can accept inputs (parameters) and return outputs (values). Functions help in reducing code repetition and improving code readability.
Why Use Functions?
- Code Reusability: Write a function once and reuse it multiple times.
- Organization: Break down complex code into smaller, manageable pieces.
- Maintainability: Easier to update and debug code when it’s organized into functions.
Creating Functions
Function Declarations
A function declaration defines a function using the function
keyword followed by the function name, a list of parameters in parentheses, and the function body enclosed in curly braces.
Syntax
function functionName(parameters) {
// Code to be executed
}
Example
function greet(name) {
console.log("Hello, " + name + "!");
}
greet("Alice"); // Output: Hello, Alice!
greet("Bob"); // Output: Hello, Bob!
In the example above, the greet
function takes a single parameter name
and logs a greeting message to the console.
Function Expressions
A function expression creates a function and assigns it to a variable. This type of function can be named or anonymous.
Syntax
let functionName = function(parameters) {
// Code to be executed
};
Example
let add = function(a, b) {
return a + b;
};
console.log(add(5, 3)); // Output: 8
Here, the add
function is defined as an anonymous function assigned to the variable add
. It adds two numbers and returns the result.
Arrow Functions
Arrow functions provide a shorter syntax for writing function expressions. They are particularly useful for writing concise functions and for handling this
keyword behavior.
Syntax
let functionName = (parameters) => {
// Code to be executed
};
Example
let multiply = (x, y) => x * y;
console.log(multiply(4, 5)); // Output: 20
In this example, the multiply
function is written using the arrow function syntax. It multiplies two numbers and returns the result. Notice that if the function has only one expression, you can omit the curly braces and the return
keyword.
The Function
Constructor
The Function
constructor creates a new function object. This method is less commonly used but provides a way to create functions dynamically.
Syntax
let functionName = new Function('parameters', 'code');
Example
let divide = new Function('a', 'b', 'return a / b;');
console.log(divide(10, 2)); // Output: 5
In this example, the divide
function is created using the Function
constructor and performs division.
Invoking Functions
Calling Functions
To execute a function, you use its name followed by parentheses. If the function has parameters, you pass the arguments inside the parentheses.
Syntax
functionName(arguments);
Example
function greet(name) {
console.log("Hello, " + name + "!");
}
greet("Charlie"); // Output: Hello, Charlie!
Function Parameters
Parameters are variables listed as part of the function definition. Arguments are the actual values passed to the function when it is called.
Example
function sum(a, b) {
return a + b;
}
console.log(sum(2, 3)); // Output: 5
In this example, a
and b
are parameters, while 2
and 3
are arguments passed to the function.
Default Parameters
JavaScript allows you to set default values for parameters in case no arguments are provided.
function greet(name = "Guest") {
console.log("Hello, " + name + "!");
}
greet(); // Output: Hello, Guest!
greet("Daisy"); // Output: Hello, Daisy!
Return Values
Functions can return values using the return
keyword. If no return
statement is provided, the function returns undefined
by default.
Example
function square(x) {
return x * x;
}
let result = square(4);
console.log(result); // Output: 16
In this example, the square
function returns the square of the input value, and the result is logged to the console.
Function Scope and Closures
Local vs. Global Scope
Variables declared inside a function are local to that function and are not accessible outside of it. Conversely, variables declared outside of any function are global and can be accessed from any function.
Example
let globalVar = "I'm global";
function example() {
let localVar = "I'm local";
console.log(globalVar); // Output: I'm global
console.log(localVar); // Output: I'm local
}
example();
console.log(globalVar); // Output: I'm global
console.log(localVar); // Error: localVar is not defined
In this example, globalVar
is accessible both inside and outside the example
function, while localVar
is only accessible inside the function.
Closures
A closure is a feature where an inner function retains access to the variables of its outer function even after the outer function has finished executing. Closures are useful for creating private variables and functions.
Example
function outerFunction() {
let outerVar = "I'm from outer function";
function innerFunction() {
console.log(outerVar);
}
return innerFunction;
}
let closure = outerFunction();
closure(); // Output: I'm from outer function
In this example, innerFunction
retains access to outerVar
even after outerFunction
has returned.
Advanced Function Concepts
Higher-Order Functions
Higher-order functions are functions that take other functions as arguments or return functions as their result. They are a key concept in functional programming.
Example
function createMultiplier(multiplier) {
return function(x) {
return x * multiplier;
};
}
let double = createMultiplier(2);
console.log(double(5)); // Output: 10
In this example, createMultiplier
returns a function that multiplies its input by the given multiplier.
Callback Functions
A callback function is a function passed as an argument to another function and is executed after the completion of that function.
Example
function processUserInput(callback) {
let name = prompt("Enter your name:");
callback(name);
}
function greet(name) {
console.log("Hello, " + name + "!");
}
processUserInput(greet);
In this example, greet
is a callback function passed to processUserInput
. It is executed after the user provides input.
Function Currying
Function currying is a technique where a function with multiple arguments is transformed into a sequence of functions, each taking a single argument.
Example
function multiply(a) {
return function(b) {
return a * b;
};
}
let double = multiply(2);
console.log(double(5)); // Output: 10
In this example, multiply
is curried into a sequence of functions that multiply a number by a
.
IIFE (Immediately Invoked Function Expressions)
An IIFE is a function that is defined and executed immediately. It is used to create a local scope and avoid polluting the global namespace.
Example
(function() {
let message = "Hello from IIFE";
console.log(message);
})();
console.log(message); // Error: message is not defined
In this example, the IIFE creates a local scope for message
, which is not accessible outside the function.
Best Practices for Functions
- Use Descriptive Names: Name functions and parameters descriptively to indicate their purpose.
- Keep Functions Small: Aim to keep functions focused on a single task or responsibility.
- Avoid Side Effects: Functions should avoid modifying external state or variables unless necessary.
- Use Default Parameters: Provide default values for parameters when appropriate to make functions more flexible.
- Document Functions: Use comments or documentation to describe the purpose, parameters, and return values of functions.
Common Pitfalls and Debugging Tips
- Incorrect
this
Context: Arrow functions do not have their ownthis
context. Use regular functions if you need to bindthis
. - Forget to Return: Ensure that functions return values as expected, especially when using them in expressions.
- Overcomplicating Functions: Avoid writing overly complex functions. Break them down into smaller, manageable pieces if needed.
- Handling Undefined Parameters: Be cautious when using parameters that may be undefined or null. Use default values or validation as necessary.
Conclusion
Functions are a cornerstone of JavaScript programming, providing a powerful way to encapsulate and reuse code. By understanding how to create and use functions effectively, you can write cleaner, more maintainable code. From basic function declarations to advanced concepts like closures and currying, mastering functions will significantly enhance your JavaScript skills.
By following best practices, avoiding common pitfalls, and leveraging advanced techniques, you can make the most out of functions and write more efficient and elegant JavaScript code. Continue to explore and experiment with functions to deepen your understanding and improve your programming expertise.