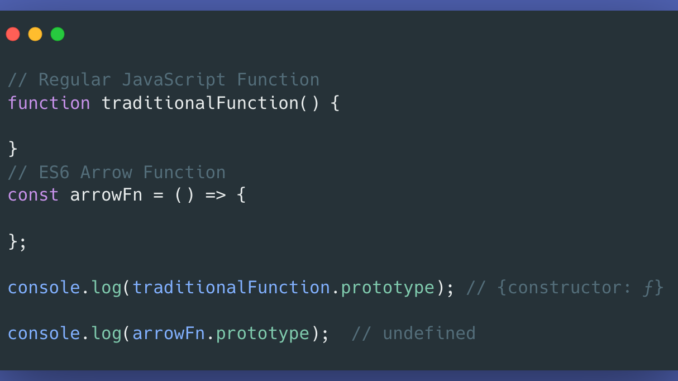
Arrow functions, introduced in ECMAScript 6 (ES6), are a concise way to write function expressions in JavaScript. They offer a shorter syntax compared to traditional function expressions and bring some unique features, such as lexical scoping of the this
keyword. This comprehensive guide will explore the syntax, features, and best practices for using arrow functions effectively in JavaScript.
Table of Contents
- Introduction to Arrow Functions
- Syntax and Basic Usage
- Single Expression Functions
- Multiple Expressions Functions
- Parameters and Argument Handling
- Key Features of Arrow Functions
- Lexical
this
Binding - No
arguments
Object - No
new
Keyword - No
prototype
Property
- Lexical
- Arrow Functions vs. Traditional Functions
- Syntax Comparison
- Behavior Comparison
- Common Use Cases for Arrow Functions
- Callback Functions
- Array Methods:
map
,filter
, andreduce
- Event Handlers
- Best Practices
- Common Pitfalls and Troubleshooting
- Advanced Arrow Function Patterns
- Chaining Arrow Functions
- Using Arrow Functions in Object Methods
- Combining Arrow Functions with Other ES6 Features
- Conclusion
Introduction to Arrow Functions
Arrow functions are a syntactically compact alternative to traditional function expressions. They are especially useful in scenarios where a concise function definition is beneficial, such as in callbacks or array operations.
Why Use Arrow Functions?
- Conciseness: They reduce the verbosity of function expressions.
- Lexical Scoping: They handle the
this
keyword differently, making them more predictable in certain contexts.
Syntax and Basic Usage
Single Expression Functions
If an arrow function consists of a single expression, you can omit the curly braces {}
and the return
keyword. The result of the expression is automatically returned.
Syntax
const functionName = (parameters) => expression;
Example
const add = (a, b) => a + b;
console.log(add(5, 3)); // Output: 8
In this example, add
is an arrow function that takes two parameters and returns their sum.
Multiple Expressions Functions
If an arrow function has multiple expressions or statements, you need to use curly braces {}
and explicitly use the return
keyword.
Syntax
const functionName = (parameters) => {
// Multiple statements
return value;
};
Example
const multiplyAndSquare = (x, y) => {
let product = x * y;
return product * product;
};
console.log(multiplyAndSquare(2, 3)); // Output: 36
Here, multiplyAndSquare
is an arrow function with multiple statements, where the result is computed and returned explicitly.
Parameters and Argument Handling
Arrow functions handle parameters similarly to traditional functions. You can have optional parameters, default values, and rest parameters.
Optional Parameters
const greet = (name = "Guest") => `Hello, ${name}!`;
console.log(greet()); // Output: Hello, Guest!
console.log(greet("Alice"));// Output: Hello, Alice!
Rest Parameters
const sum = (...numbers) => numbers.reduce((acc, num) => acc + num, 0);
console.log(sum(1, 2, 3, 4)); // Output: 10
In this example, sum
uses rest parameters to accept any number of arguments and calculates their sum.
Key Features of Arrow Functions
Lexical this
Binding
One of the main differences between arrow functions and traditional functions is that arrow functions do not have their own this
context. Instead, they inherit this
from the enclosing lexical context. This can be particularly useful in scenarios involving callbacks and event handlers.
Example
function Timer() {
this.seconds = 0;
setInterval(() => {
this.seconds++;
console.log(this.seconds);
}, 1000);
}
new Timer();
In this example, the arrow function inside setInterval
inherits this
from the Timer
function, so this.seconds
correctly refers to the seconds
property of the Timer
instance.
No arguments
Object
Arrow functions do not have their own arguments
object. If you need to access arguments, you can use rest parameters.
Example
function showArguments() {
const arrowFunc = () => {
console.log(arguments); // ReferenceError: arguments is not defined
};
arrowFunc();
}
showArguments(1, 2, 3);
In this example, arrowFunc
does not have access to the arguments
object. You should use rest parameters or Function.prototype.apply
/Function.prototype.call
if you need similar functionality.
No new
Keyword
Arrow functions cannot be used as constructors and do not have the new
keyword.
Example
const Person = (name) => {
this.name = name;
};
// Error
let john = new Person("John");
In this example, trying to use Person
as a constructor with new
will result in an error.
No prototype
Property
Arrow functions do not have a prototype
property, which means they cannot be used with prototype-based inheritance.
Example
const func = () => {};
console.log(func.prototype); // Output: undefined
In this example, func
does not have a prototype
property, and you cannot use it for inheritance.
Arrow Functions vs. Traditional Functions
Syntax Comparison
Arrow functions provide a more concise syntax compared to traditional function expressions.
Traditional Function Expression
const add = function(a, b) {
return a + b;
};
Arrow Function
const add = (a, b) => a + b;
Behavior Comparison
this
Binding
Traditional function expressions have their own this
context, while arrow functions inherit this
from the surrounding context.
function TraditionalFunction() {
this.value = 1;
setTimeout(function() {
console.log(this.value); // Output: undefined (in strict mode)
}, 1000);
}
function ArrowFunction() {
this.value = 1;
setTimeout(() => {
console.log(this.value); // Output: 1
}, 1000);
}
In this example, TraditionalFunction
loses its this
context in the setTimeout
function, whereas ArrowFunction
retains it due to lexical scoping.
arguments
Object
Traditional functions have an arguments
object, while arrow functions do not.
function traditionalFunction() {
console.log(arguments);
}
const arrowFunction = () => {
console.log(arguments); // ReferenceError: arguments is not defined
};
In this example, traditionalFunction
can access the arguments
object, but arrowFunction
cannot.
Common Use Cases for Arrow Functions
Callback Functions
Arrow functions are commonly used for callbacks in array methods and other asynchronous operations due to their concise syntax and lexical scoping of this
.
Example with Array Methods
const numbers = [1, 2, 3, 4, 5];
const doubled = numbers.map(number => number * 2);
console.log(doubled); // Output: [2, 4, 6, 8, 10]
In this example, an arrow function is used to double each element in the numbers
array.
Event Handlers
Arrow functions are useful for event handlers, especially when you need to access properties of the enclosing context.
Example
class Counter {
constructor() {
this.count = 0;
document.querySelector('button').addEventListener('click', () => {
this.count++;
console.log(this.count);
});
}
}
new Counter();
In this example, the arrow function in the addEventListener
call retains the this
context of the Counter
instance.
Best Practices
- Use Arrow Functions for Conciseness: Utilize arrow functions when you need a concise function expression.
- Avoid Arrow Functions for Methods: Avoid using arrow functions for object methods if you need to use
this
. - Leverage Lexical
this
Binding: Use arrow functions in callbacks and event handlers where lexical scoping ofthis
is beneficial. - Handle Arguments Carefully: Use rest parameters instead of relying on the
arguments
object.
Common Pitfalls and Troubleshooting
Misunderstanding this
The behavior of this
in arrow functions can lead to confusion. Make sure to understand that arrow functions do not have their own this
and use it to capture the surrounding context.
Not Suitable for Methods
Avoid using arrow functions for object methods if you need to reference this
within the method. Instead, use traditional function expressions for methods.
Using arguments
Object
Arrow functions do not have the arguments
object. If you need to work with variable arguments, use rest parameters.
Advanced Arrow Function Patterns
Chaining Arrow Functions
You can chain multiple arrow functions together for complex transformations.
Example
const processNumbers = numbers => numbers
.filter(n => n % 2 === 0)
.map(n => n * 2)
.reduce((acc, n) => acc + n, 0);
console.log(processNumbers([1, 2, 3, 4, 5])); // Output: 12
In this example, arrow functions are chained to filter, map, and reduce an array of numbers.
Using Arrow Functions in Object Methods
If you need to use an arrow function within an object but still want to access this
, consider using a regular function or ensuring proper context.
Example
const obj = {
name: 'Alice',
greet: function() {
setTimeout(() => {
console.log(`Hello, ${this.name}!`);
}, 1000);
}
};
obj.greet(); // Output: Hello, Alice!
Here, the arrow function inside setTimeout
captures the this
context of greet
.
Combining Arrow Functions with Other ES6 Features
Arrow functions work well with other ES6 features such as destructuring, template literals, and spread operators.
Example with Destructuring
const greet = ({ name }) => `Hello, ${name}!`;
console.log(greet({ name: 'Bob' })); // Output: Hello, Bob!
In this example, destructuring is used in the parameter list of an arrow function.
Conclusion
Arrow functions in JavaScript provide a more concise syntax for defining functions and introduce unique features such as lexical scoping of this
. Understanding how to create and use arrow functions effectively can help you write cleaner, more efficient code.
By exploring their syntax, features, and best practices, you can leverage arrow functions to enhance your JavaScript programming skills. Whether you use them for callbacks, array methods, or other functional programming tasks, mastering arrow functions will make you a more proficient and versatile developer.