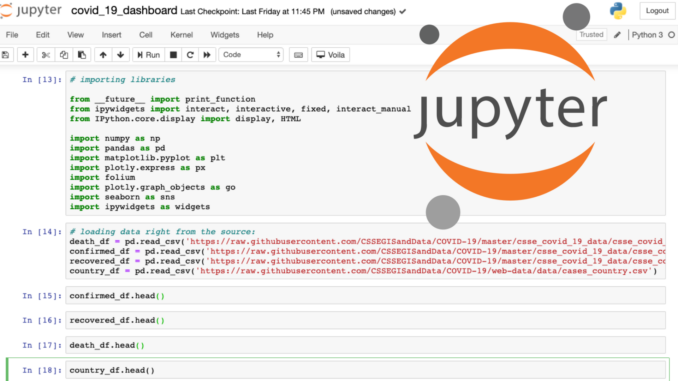
Introduction
Jupyter Notebooks have revolutionized the way we write and share code in Python, offering an interactive and versatile environment ideal for data analysis, scientific research, and educational purposes. With its ability to combine executable code, rich text, visualizations, and more in a single document, Jupyter Notebooks have become a standard tool for many Python users.
In this comprehensive guide, we will explore the ins and outs of Jupyter Notebooks, covering everything from installation and setup to advanced usage and best practices. Whether you’re a beginner or an experienced Python programmer, this guide will help you harness the full power of Jupyter Notebooks for your projects.
Table of Contents
- What is Jupyter Notebook?
- Installing Jupyter Notebook
- Using Anaconda
- Using pip
- Getting Started with Jupyter Notebook
- Launching Jupyter Notebook
- Understanding the Interface
- Writing and Executing Code in Jupyter Notebooks
- Creating Cells
- Writing Python Code
- Running Cells
- Adding Rich Text and Markdown
- Using Markdown
- Adding LaTeX Equations
- Visualizing Data in Jupyter Notebooks
- Basic Plotting with Matplotlib
- Advanced Visualizations with Seaborn
- Interactive Plots with Plotly
- Importing and Exporting Notebooks
- Exporting to HTML, PDF, and Other Formats
- Importing Notebooks
- Advanced Features and Extensions
- Using Magic Commands
- Integrating with JupyterLab
- Installing and Using Extensions
- Best Practices for Using Jupyter Notebooks
- Organizing Your Notebooks
- Version Control
- Collaborating with Others
- Troubleshooting Common Issues
- Conclusion
What is Jupyter Notebook?
Jupyter Notebook is an open-source web application that allows you to create and share documents containing live code, equations, visualizations, and narrative text. It supports interactive data science and scientific computing across multiple programming languages, although it is most commonly used with Python.
Key features of Jupyter Notebooks include:
- Interactive Code Execution: Run code in real-time and see immediate results.
- Rich Text Support: Use Markdown and LaTeX to format text, create headings, and include mathematical equations.
- Data Visualization: Integrate with libraries like Matplotlib, Seaborn, and Plotly to create plots and visualizations.
- Notebook Sharing: Share your notebooks with others through platforms like GitHub or JupyterHub.
Installing Jupyter Notebook
Before you can start using Jupyter Notebooks, you need to install them on your system. There are two common methods for installation: using Anaconda or using pip.
Using Anaconda
Anaconda is a popular distribution that includes Jupyter Notebook along with other data science packages. If you don’t already have Anaconda installed, follow these steps:
- Download Anaconda: Go to the Anaconda website and download the Anaconda distribution for your operating system.
- Run the Installer: Open the downloaded installer file and follow the instructions to complete the installation.
- Launch Jupyter Notebook: Open the Anaconda Navigator application and click on the “Launch” button under Jupyter Notebook. Alternatively, you can open a terminal or command prompt and type
jupyter notebook
to start the notebook server.
Using pip
If you prefer not to use Anaconda, you can install Jupyter Notebook using pip, Python’s package installer.
- Install Jupyter Notebook: Open a terminal or command prompt and run the following command:
sh
pip install notebook
- Launch Jupyter Notebook: After installation, start the Jupyter Notebook server by typing:
sh
jupyter notebook
This command will open a new tab in your web browser with the Jupyter Notebook interface.
Getting Started with Jupyter Notebook
Once Jupyter Notebook is installed, you’ll want to get familiar with the interface and basic operations.
Launching Jupyter Notebook
To launch Jupyter Notebook, use the following command in your terminal or command prompt:
jupyter notebook
This command starts the Jupyter Notebook server and opens the Notebook Dashboard in your default web browser. The Dashboard is the main interface where you can manage and open your notebooks.
Understanding the Interface
The Jupyter Notebook interface consists of several key components:
- Notebook Dashboard: Displays a list of notebooks and files in the current directory. You can navigate directories, create new notebooks, and open existing ones from here.
- Notebook: The main area where you write and execute code. Each notebook is made up of cells, which can contain code, Markdown text, or other content.
- Menu Bar: Located at the top of the notebook, the menu bar provides options for saving, opening, and editing notebooks, as well as running code and accessing tools.
- Toolbar: Below the menu bar, the toolbar contains buttons for common actions such as saving the notebook, running cells, and adding new cells.
Writing and Executing Code in Jupyter Notebooks
The core functionality of Jupyter Notebooks revolves around writing and executing code. Here’s how to get started with code cells:
Creating Cells
Cells are the building blocks of Jupyter Notebooks. There are different types of cells, but the most common are code cells and Markdown cells.
- Adding a New Cell: To add a new cell, click on the “Insert” menu and select “Insert Cell Above” or “Insert Cell Below”. Alternatively, you can use the
+
button in the toolbar. - Cell Types: By default, new cells are code cells. You can change a cell’s type by selecting it and choosing a different type from the dropdown menu in the toolbar or using keyboard shortcuts.
Writing Python Code
To write code in a cell, simply click inside the cell and start typing. Here’s an example of basic Python code in a code cell:
# This is a code cell
import math
def calculate_area(radius):
return math.pi * radius ** 2
radius = 5
area = calculate_area(radius)
print(f"The area of the circle with radius {radius} is {area:.2f}")
Running Cells
To execute the code in a cell, select the cell and press Shift + Enter
, or click the “Run” button in the toolbar. The output of the code will be displayed directly below the cell.
- Running All Cells: To run all cells in the notebook, select “Cell” from the menu bar and choose “Run All”.
- Stopping Execution: If you need to interrupt a running cell, click on “Kernel” in the menu bar and select “Interrupt”.
Adding Rich Text and Markdown
Jupyter Notebooks allow you to include rich text and formatted content using Markdown. This is useful for adding explanations, notes, and documentation alongside your code.
Using Markdown
Markdown is a lightweight markup language that you can use to format text in your notebooks. You can use Markdown cells to include headings, lists, links, images, and more.
- Creating a Markdown Cell: Add a new cell and change its type to “Markdown” from the dropdown menu or toolbar.
- Writing Markdown: Enter Markdown text into the cell. For example:
markdown
# Heading 1
## Heading 2
### Heading 3**Bold Text**
*Italic Text*
- List item 1
- List item 2[Link to Google](https://www.google.com)
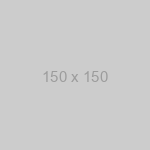
- Rendering Markdown: Run the Markdown cell by pressing
Shift + Enter
to see the formatted text.
Adding LaTeX Equations
LaTeX is a typesetting system commonly used for mathematical and scientific documents. You can include LaTeX equations in Markdown cells by enclosing the LaTeX code within dollar signs.
- Inline Equations: Use single dollar signs for inline equations. For example:
$E = mc^2$
. - Block Equations: Use double dollar signs for block equations. For example:
markdown
$$
\frac{d}{dx} \left( \int_{a}^{b} f(x) \, dx \right) = f(x)
$$
Visualizing Data in Jupyter Notebooks
Data visualization is a crucial aspect of data analysis. Jupyter Notebooks support various libraries for creating plots and visualizations.
Basic Plotting with Matplotlib
Matplotlib is a popular plotting library in Python. You can create a wide range of plots, including line plots, bar plots, and histograms.
- Importing Matplotlib:
python
import matplotlib.pyplot as plt
- Creating a Simple Plot:
python
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]plt.plot(x, y)
plt.xlabel('X Axis')
plt.ylabel('Y Axis')
plt.title('Simple Line Plot')
plt.show()
Advanced Visualizations with Seaborn
Seaborn is built on top of Matplotlib and provides a higher-level interface for creating attractive and informative statistical graphics.
- Importing Seaborn:
python
import seaborn as sns
import pandas as pd
- Creating a Seaborn Plot:
python
# Sample DataFrame
data = pd.DataFrame({
'x': [1, 2, 3, 4, 5],
'y': [2, 4, 6, 8, 10],
'category': ['A', 'B', 'A', 'B', 'A']
})sns.scatterplot(data=data, x='x', y='y', hue='category')
plt.title('Scatter Plot with Seaborn')
plt.show()
Interactive Plots with Plotly
Plotly is a library for creating interactive plots. It allows users to hover over, zoom, and pan within the plots.
- Installing Plotly:
sh
pip install plotly
- Creating an Interactive Plot:
python
import plotly.express as px
# Sample DataFrame
data = pd.DataFrame({
'x': [1, 2, 3, 4, 5],
'y': [2, 4, 6, 8, 10],
'category': ['A', 'B', 'A', 'B', 'A']
})fig = px.scatter(data, x='x', y='y', color='category', title='Interactive Scatter Plot')
fig.show()
Importing and Exporting Notebooks
Jupyter Notebooks can be exported to various formats, making it easy to share your work or convert it to different document types.
Exporting to HTML, PDF, and Other Formats
- Exporting from the Notebook Interface:
- Open the notebook you want to export.
- Go to the “File” menu, select “Download as”, and choose the desired format (e.g., HTML, PDF).
- Exporting via Command Line:
Use the
nbconvert
command to export notebooks from the command line:shjupyter nbconvert --to html notebook.ipynb
jupyter nbconvert --to pdf notebook.ipynb
Importing Notebooks
To open a notebook, use the Notebook Dashboard to navigate to the file and click on it. You can also import notebooks programmatically using the nbformat
library.
import nbformat
with open('notebook.ipynb') as f:
notebook = nbformat.read(f, as_version=4)
Advanced Features and Extensions
Jupyter Notebooks offer several advanced features and extensions that enhance functionality and usability.
Using Magic Commands
Magic commands are special commands in Jupyter that start with %
or %%
and provide additional functionality.
- Line Magics (Single
%
):python%timeit [x**2 for x in range(1000)] # Measure execution time
- Cell Magics (Double
%%
):python%%capture
# This cell's output will be suppressed
print("This will not be displayed")
Integrating with JupyterLab
JupyterLab is the next-generation interface for Jupyter, offering a more flexible and powerful environment compared to the classic Notebook interface.
- Installing JupyterLab:
sh
pip install jupyterlab
- Launching JupyterLab:
sh
jupyter lab
JupyterLab provides a modern, tabbed interface where you can work with notebooks, text editors, terminals, and more in a unified workspace.
Installing and Using Extensions
Jupyter Notebooks support various extensions that enhance functionality. You can install and manage extensions using the jupyter_contrib_nbextensions
package.
- Installing Jupyter Extensions:
sh
pip install jupyter_contrib_nbextensions
jupyter contrib nbextension install --user
- Enabling Extensions:
- Open a notebook and go to the “Nbextensions” tab.
- Browse the available extensions and enable the ones you need.
Best Practices for Using Jupyter Notebooks
To make the most of Jupyter Notebooks, follow these best practices:
Organizing Your Notebooks
- Use Clear Titles and Descriptions: Clearly title your notebooks and provide descriptions for sections using Markdown cells.
- Modularize Code: Break your code into smaller, manageable cells to make it easier to understand and debug.
- Include Documentation: Add Markdown cells to explain your code, describe your analysis, and document your findings.
Version Control
- Use Git for Version Control: Track changes to your notebooks and collaborate with others using Git. Store your notebooks in a Git repository for version control.
- Convert to Script: Use
nbconvert
to convert notebooks to Python scripts if needed:shjupyter nbconvert --to script notebook.ipynb
Collaborating with Others
- Share Notebooks: Share your notebooks with others by exporting them to formats like HTML or PDF or by hosting them on platforms like GitHub.
- Use JupyterHub: For collaborative environments, JupyterHub allows multiple users to work with Jupyter Notebooks on a shared server.
Troubleshooting Common Issues
While using Jupyter Notebooks, you may encounter some common issues. Here are solutions to help you troubleshoot:
Common Issues
- Kernel Not Starting: If the kernel fails to start, try restarting Jupyter Notebook or reinstalling Jupyter. Ensure that the Python environment is correctly set up.
- Code Not Executing: Check for syntax errors in your code or missing dependencies. Ensure that all required packages are installed.
- File Not Found: Make sure that the file paths specified in your code are correct and that the files exist in the specified locations.
Debugging Tips
- Check Error Messages: Carefully read any error messages displayed in the notebook. They often provide clues about what went wrong and where to look.
- Restart the Kernel: Sometimes restarting the kernel can resolve issues related to memory or state. Use “Kernel” > “Restart” from the menu.
- Clear Output: Clear the output of cells by selecting “Cell” > “All Output” > “Clear” to resolve issues related to outdated or conflicting output.
Conclusion
Jupyter Notebooks are a powerful and versatile tool for Python programming, offering an interactive environment that combines code, rich text, and visualizations in a single document. By following this comprehensive guide, you have learned how to install, use, and extend Jupyter Notebooks, as well as best practices for effective usage. Whether you’re conducting data analysis, writing scientific research, or teaching Python, Jupyter Notebooks provide a flexible and user-friendly platform to support your work. Happy coding and exploring with Jupyter Notebooks!