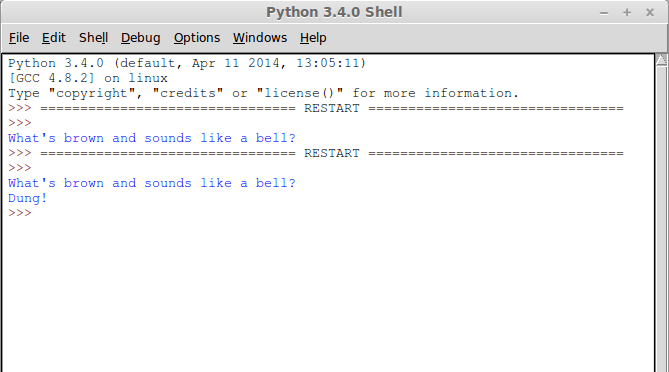
Introduction
Python IDLE (Integrated Development and Learning Environment) is a simple and lightweight IDE that comes bundled with the Python installation package. It is designed specifically for Python development and is a great tool for beginners and experienced developers alike. IDLE provides a user-friendly interface with features like syntax highlighting, code completion, and an interactive shell. This comprehensive guide will walk you through the basics of using Python IDLE, from installation to advanced usage. By the end of this guide, you will have a solid understanding of how to leverage IDLE for your Python programming needs.
Table of Contents
- What is Python IDLE?
- Installing Python and IDLE
- Getting Started with IDLE
- Writing and Running Python Code in IDLE
- Exploring IDLE Features
- Interactive Shell
- Script Editor
- Syntax Highlighting
- Code Completion
- Debugger
- Configuration Options
- Advanced IDLE Usage
- Using Modules and Packages
- Virtual Environments in IDLE
- Integrating with Version Control
- Troubleshooting and Common Issues
- Tips and Best Practices
- Conclusion
What is Python IDLE?
Python IDLE is an Integrated Development Environment (IDE) that comes pre-installed with Python. It is a simple, lightweight tool that provides an easy way to write, run, and debug Python programs. IDLE is particularly well-suited for beginners due to its straightforward interface and ease of use. Despite its simplicity, IDLE includes many features found in more advanced IDEs, such as:
- Interactive Shell: Allows you to execute Python commands one at a time and see immediate results.
- Script Editor: Provides a space to write and save complete Python programs.
- Syntax Highlighting: Colors different parts of your code to make it easier to read and understand.
- Code Completion: Suggests possible completions for partially typed code.
- Debugger: Helps you find and fix errors in your code.
- Configuration Options: Allows you to customize the appearance and behavior of IDLE.
Installing Python and IDLE
Before you can use IDLE, you need to have Python installed on your system. Python IDLE is included with the standard Python distribution, so installing Python will also install IDLE.
Windows
- Download Python: Go to the official Python website at python.org and download the Windows installer.
- Run the Installer: Open the downloaded file and follow the prompts. Make sure to check the box that says “Add Python to PATH” before clicking Install.
- Verify Installation: Open the Command Prompt and type
python --version
to ensure Python is installed correctly. You can also typeidle
to open IDLE.
macOS
- Download Python: Visit the python.org website and download the macOS installer.
- Run the Installer: Open the downloaded file and follow the instructions to install Python.
- Verify Installation: Open Terminal and type
python3 --version
to verify the installation. You can also typeidle3
to open IDLE.
Linux
Most Linux distributions come with Python pre-installed. However, you might need to install IDLE separately.
- Install Python and IDLE: Open Terminal and type the following command:
sh
sudo apt-get install python3 python3-idle
- Verify Installation: Type
python3 --version
to check the Python version andidle3
to open IDLE.
Getting Started with IDLE
Once you have Python and IDLE installed, you are ready to start using IDLE. This section will guide you through the basics of opening IDLE, navigating the interface, and understanding the key components.
Opening IDLE
To open IDLE, follow these steps:
- Windows: Open the Start Menu, type “IDLE” in the search bar, and select “IDLE (Python 3.x.x)”.
- macOS: Open Spotlight (Cmd + Space), type “IDLE”, and select “IDLE (Python 3.x.x)”.
- Linux: Open Terminal and type
idle3
.
Navigating the Interface
When you first open IDLE, you will see the Python Shell window. This is an interactive environment where you can type Python commands and see immediate results. The IDLE interface consists of the following key components:
- Menu Bar: Located at the top, it provides access to various options and settings, such as File, Edit, Format, Run, Debug, Options, and Help.
- Python Shell: The main area where you can enter and execute Python commands interactively.
- Editor Window: Opens when you create a new file or open an existing file. This is where you write and edit complete Python scripts.
Writing and Running Python Code in IDLE
This section will guide you through the process of writing and running Python code in IDLE. We will start with simple commands in the Python Shell and then move on to writing and executing complete scripts in the Editor Window.
Using the Python Shell
The Python Shell is an interactive environment where you can enter Python commands and see immediate results. This is a great place to experiment with Python code and test small snippets.
- Simple Commands: Type the following commands one at a time and press Enter to see the results:
python
print("Hello, World!")
2 + 2
x = 10
x * 2
- Using Functions: Define and call a simple function:
python
def greet(name):
return "Hello, " + name + "!"greet("Alice")
- Exploring Built-in Functions: Use some built-in functions:
python
len("Hello")
type(42)
Writing and Running Scripts
While the Python Shell is useful for interactive experimentation, you will often need to write and run complete scripts. This is where the Editor Window comes in.
- Creating a New Script:
- Open a new Editor Window by selecting
File > New File
from the menu bar. - Write a simple Python script. For example:
python
# This is a simple Python script
name = input("Enter your name: ")
print("Hello, " + name + "!")
- Open a new Editor Window by selecting
- Saving Your Script:
- Save your script by selecting
File > Save
from the menu bar (or pressCtrl+S
). - Choose a location and enter a filename with a
.py
extension, such asgreet.py
.
- Save your script by selecting
- Running Your Script:
- Run your script by selecting
Run > Run Module
from the menu bar (or pressF5
). - The script will execute, and you will see the output in the Python Shell.
- Run your script by selecting
Exploring IDLE Features
IDLE comes with several features that enhance your coding experience. This section will explore some of the key features and how to use them effectively.
Interactive Shell
The interactive shell is a powerful tool for testing and debugging code snippets. You can enter Python commands one at a time and see immediate results. The shell supports all Python statements, including loops, conditionals, and function definitions.
- Using Loops and Conditionals:
python
for i in range(5):
print("Iteration:", i)age = int(input("Enter your age: "))
if age < 18:
print("You are a minor.")
else:
print("You are an adult.")
- Defining and Using Functions:
python
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n-1)factorial(5)
Script Editor
The script editor allows you to write and save complete Python programs. It provides features like syntax highlighting, code completion, and error checking to enhance your coding experience.
- Syntax Highlighting: Different parts of your code, such as keywords, strings, and comments, are colored differently to make the code easier to read.
- Code Completion: As you type, IDLE suggests possible completions for partially typed words. You can select a suggestion by pressing Tab or Enter.
- Error Checking: IDLE underlines syntax errors in red, helping you identify and fix mistakes quickly.
Debugger
IDLE includes a built-in debugger that helps you find and fix errors in your code. The debugger allows you to set breakpoints, step through your code, and inspect variables.
- Setting Breakpoints: Click in the left margin of the Editor Window to set a breakpoint. A red dot will appear, indicating the breakpoint.
- Starting the Debugger: Run your script in debug mode by selecting
Debug > Debugger
from the menu bar and thenRun > Run Module
(or pressF5
). - Stepping Through Code: Use the buttons in the Debug Control window to step through your code line by line. You can inspect variables and see the flow of execution.
Configuration Options
IDLE allows you to customize the appearance and behavior of the interface. You can access the configuration options by selecting Options > Configure IDLE
from the menu bar.
- Fonts and Colors: Change the font type, size, and color scheme used in the Editor Window and Python Shell.
- General Settings: Configure options like autosave, indentation settings, and startup options.
- Key Bindings: Customize the keyboard shortcuts used in IDLE.
Advanced IDLE Usage
Beyond the basics, IDLE offers features that allow for more advanced usage, such as working with modules and packages, setting up virtual environments, and integrating with version control systems.
Using Modules and Packages
Modules and packages are essential for organizing and reusing code. IDLE makes it easy to work with them.
- Importing Modules: Import built-in and third-party modules in your scripts:
python
import math
print(math.sqrt(16))import requests
response = requests.get("https://www.python.org")
print(response.status_code)
- Creating Your Own Modules: Save reusable functions in a separate file and import them in your scripts:
python
# Save this as mymodule.py
def greet(name):
return "Hello, " + name + "!"# Import and use in another script
import mymodule
print(mymodule.greet("Alice"))
Virtual Environments in IDLE
Virtual environments allow you to create isolated Python environments with their own dependencies. This is useful for managing project-specific libraries and avoiding conflicts.
- Creating a Virtual Environment:
sh
python -m venv myenv
- Activating the Virtual Environment:
- Windows:
myenv\Scripts\activate
- macOS/Linux:
source myenv/bin/activate
- Windows:
- Using the Virtual Environment in IDLE: Open IDLE from within the activated virtual environment to ensure it uses the correct Python interpreter and libraries.
Integrating with Version Control
Version control systems like Git help you track changes to your code and collaborate with others. You can integrate IDLE with version control tools.
- Installing Git: Download and install Git from git-scm.com.
- Initializing a Repository: Open Terminal and navigate to your project directory. Run the following command:
sh
git init
- Committing Changes: Add and commit your changes:
sh
git add .
git commit -m "Initial commit"
- Using Git with IDLE: Use the Terminal within IDLE to run Git commands and manage your repository.
Troubleshooting and Common Issues
While using IDLE, you may encounter some common issues. This section provides solutions to help you troubleshoot and resolve these problems.
Common Issues
- IDLE Not Opening: If IDLE does not open, ensure Python is installed correctly and added to your PATH. Reinstall Python if necessary.
- Syntax Errors: Check your code for missing or misplaced punctuation, incorrect indentation, and other syntax mistakes.
- Module Not Found: Ensure the module is installed and accessible. Use
pip install module_name
to install missing modules.
Debugging Tips
- Use Print Statements: Insert
print
statements to check the values of variables and the flow of execution. - Read Error Messages: Carefully read error messages to understand what went wrong and where the error occurred.
- Use the Debugger: Set breakpoints and step through your code to identify and fix issues.
Tips and Best Practices
To get the most out of IDLE and improve your Python coding experience, consider the following tips and best practices:
- Write Clear and Readable Code: Use meaningful variable names, consistent indentation, and comments to make your code easy to understand.
- Keep Your Code Organized: Use functions, modules, and packages to organize your code and make it reusable.
- Test Your Code: Write test cases to ensure your code works as expected. Use print statements and the debugger to identify and fix issues.
- Stay Updated: Keep your Python installation and IDLE up to date to benefit from the latest features and improvements.
- Explore Documentation: Refer to the official Python documentation and IDLE help resources to learn more about available features and best practices.
Conclusion
Python IDLE is a powerful and user-friendly IDE that is perfect for beginners and experienced developers alike. With its interactive shell, script editor, syntax highlighting, code completion, and debugging tools, IDLE provides everything you need to write, run, and debug Python code efficiently. By following this comprehensive guide, you have learned how to install and use IDLE, write and run Python programs, explore advanced features, and troubleshoot common issues. With practice and continued learning, you can harness the full potential of Python and IDLE to create amazing projects and become a proficient Python programmer. Happy coding!