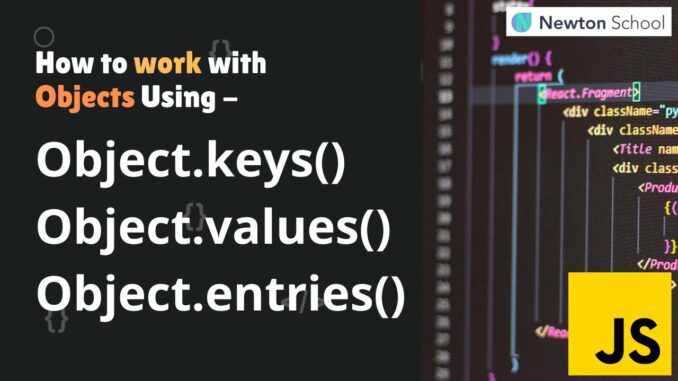
JavaScript is an object-oriented programming language where objects play a central role. Understanding how to work with objects effectively is essential for any JavaScript developer. Objects in JavaScript allow you to group related data and functions, providing a flexible way to represent and manipulate complex data structures.
This comprehensive guide covers everything you need to know about working with objects in JavaScript, including their creation, manipulation, and advanced techniques. We will explore the following topics:
- Introduction to JavaScript Objects
- Creating Objects
- Object Literals
- Constructor Functions
- ES6 Classes
- Object.create() Method
- Accessing and Modifying Object Properties
- Dot Notation
- Bracket Notation
- Computed Property Names
- Methods and
this
Keyword- Defining Methods
- Understanding
this
- Arrow Functions and
this
- Prototypes and Inheritance
- Prototype Chain
- Constructor Functions and Prototypes
- ES6 Classes and Inheritance
- Object Destructuring
- Object Methods and Utilities
- Object.assign()
- Object.keys()
- Object.values()
- Object.entries()
- Object.freeze() and Object.seal()
- Advanced Object Patterns
- Factory Functions
- Singleton Pattern
- Module Pattern
- Common Pitfalls and Debugging Tips
- Best Practices
- Conclusion
Introduction to JavaScript Objects
In JavaScript, an object is a collection of properties, where each property is a key-value pair. Objects can store various types of values, including primitive types, arrays, and even other objects. They provide a way to model real-world entities and manage related data.
Why Use Objects?
- Encapsulation: Objects allow you to bundle related data and functions together.
- Flexibility: Objects can be easily extended and modified.
- Real-World Modeling: Objects are ideal for representing complex entities like users, products, and more.
Creating Objects
Object Literals
The simplest way to create an object is by using an object literal. This method allows you to define an object with a set of key-value pairs.
Syntax
let objectName = {
key1: value1,
key2: value2,
// More properties
};
Example
let car = {
make: 'Toyota',
model: 'Camry',
year: 2020,
start: function() {
console.log('Car started');
}
};
console.log(car.make); // Output: Toyota
car.start(); // Output: Car started
In this example, car
is an object with properties make
, model
, and year
, and a method start
.
Constructor Functions
Constructor functions are used to create multiple instances of similar objects. They use the new
keyword to instantiate objects.
Syntax
function ConstructorFunction(parameter1, parameter2) {
this.property1 = parameter1;
this.property2 = parameter2;
this.method = function() {
// Method code
};
}
Example
function Person(name, age) {
this.name = name;
this.age = age;
this.greet = function() {
console.log(`Hello, my name is ${this.name}`);
};
}
let person1 = new Person('Alice', 30);
let person2 = new Person('Bob', 25);
person1.greet(); // Output: Hello, my name is Alice
person2.greet(); // Output: Hello, my name is Bob
Here, Person
is a constructor function that creates person objects with name
and age
properties and a greet
method.
ES6 Classes
ES6 introduced class syntax as a more concise and familiar way to create constructor functions and handle inheritance.
Syntax
class ClassName {
constructor(parameter1, parameter2) {
this.property1 = parameter1;
this.property2 = parameter2;
}
method() {
// Method code
}
}
Example
class Animal {
constructor(name) {
this.name = name;
}
speak() {
console.log(`${this.name} makes a noise.`);
}
}
class Dog extends Animal {
speak() {
console.log(`${this.name} barks.`);
}
}
let dog = new Dog('Rex');
dog.speak(); // Output: Rex barks.
In this example, Animal
is a class with a speak
method, and Dog
is a subclass that overrides the speak
method.
Object.create() Method
The Object.create()
method creates a new object with the specified prototype object and properties.
Syntax
let newObject = Object.create(proto, propertiesObject);
Example
let animal = {
eat() {
console.log('Eating');
}
};
let dog = Object.create(animal);
dog.bark = function() {
console.log('Barking');
};
dog.eat(); // Output: Eating
dog.bark(); // Output: Barking
In this example, dog
inherits the eat
method from the animal
prototype and also has its own bark
method.
Accessing and Modifying Object Properties
Dot Notation
Dot notation is the most common way to access or modify object properties. It requires you to use the exact property name.
Syntax
objectName.propertyName
Example
let person = {
name: 'John',
age: 30
};
console.log(person.name); // Output: John
person.age = 31;
console.log(person.age); // Output: 31
Bracket Notation
Bracket notation allows you to access or modify object properties using a string or variable. This method is useful when property names are dynamic or not valid identifiers.
Syntax
objectName['propertyName']
Example
let person = {
name: 'John',
age: 30
};
console.log(person['name']); // Output: John
person['age'] = 31;
console.log(person['age']); // Output: 31
Using Variables
let prop = 'name';
console.log(person[prop]); // Output: John
Computed Property Names
In ES6, you can use computed property names to dynamically set object properties.
Syntax
let object = {
[dynamicKey]: value
};
Example
let key = 'color';
let car = {
[key]: 'red'
};
console.log(car.color); // Output: red
Methods and this
Keyword
Defining Methods
Methods are functions that belong to objects. They are defined similarly to properties but include function definitions.
Example
let person = {
name: 'Alice',
greet: function() {
console.log(`Hello, my name is ${this.name}`);
}
};
person.greet(); // Output: Hello, my name is Alice
Understanding this
In JavaScript, the this
keyword refers to the object that is currently executing the code. Its value depends on the context in which a function is called.
Example
function showThis() {
console.log(this);
}
let obj = {
showThis: showThis
};
obj.showThis(); // Output: obj (the object calling the method)
In this example, this
refers to obj
because showThis
is called as a method of obj
.
Arrow Functions and this
Arrow functions do not have their own this
context. Instead, they inherit this
from the surrounding lexical scope.
Example
let obj = {
name: 'Bob',
greet: function() {
setTimeout(() => {
console.log(`Hello, ${this.name}`);
}, 1000);
}
};
obj.greet(); // Output: Hello, Bob
Here, the arrow function inside setTimeout
inherits this
from the greet
method, so it correctly refers to obj
.
Prototypes and Inheritance
Prototype Chain
In JavaScript, every object has a prototype object, and it forms a prototype chain. The prototype chain allows objects to inherit properties and methods from other objects.
Example
function Animal(name) {
this.name = name;
}
Animal.prototype.eat = function() {
console.log(`${this.name} is eating.`);
};
let dog = new Animal('Rex');
dog.eat(); // Output: Rex is eating.
In this example, Animal
has a prototype method eat
that is inherited by all instances of Animal
.
Constructor Functions and Prototypes
Constructor functions use prototypes to share methods among instances, reducing memory usage.
Example
function Person(name) {
this.name = name;
}
Person.prototype.sayHello = function() {
console.log(`Hello, my name is ${this.name}`);
};
let person1 = new Person('Alice');
let person2 = new Person('Bob');
person1.sayHello(); // Output: Hello, my name is Alice
person2.sayHello(); // Output: Hello, my name is Bob
In this example, sayHello
is shared among all instances of Person
via the prototype.
ES6 Classes and Inheritance
ES6 classes provide a more structured way to create objects and handle inheritance.
Syntax
class Animal {
constructor(name) {
this.name = name;
}
speak() {
console.log(`${this.name} makes a noise.`);
}
}
class Dog extends Animal {
speak() {
console.log(`${this.name} barks.`);
}
}
let dog = new Dog('Rex');
dog.speak(); // Output: Rex barks.
In this example, Dog
extends Animal
, inheriting its properties and methods and overriding the speak
method.
Object Destructuring
Object destructuring is a syntax that allows you to unpack values from objects into distinct variables.
Basic Destructuring
Syntax
const { property1, property2 } = objectName;
Example
const person = {
name: 'Alice',
age: 30
};
const { name, age } = person;
console.log(name); // Output: Alice
console.log(age); // Output: 30
Nested Destructuring
You can also destructure nested objects.
Example
const person = {
name: 'Alice',
address: {
city: 'New York',
zip: '10001'
}
};
const { address: { city, zip } } = person;
console.log(city); // Output: New York
console.log(zip); // Output: 10001
Default Values
Provide default values if a property is undefined.
Example
const person = {
name: 'Alice'
};
const { name, age = 30 } = person;
console.log(name); // Output: Alice
console.log(age); // Output: 30
Object Methods and Utilities
Object.assign()
The Object.assign()
method copies the values of all enumerable properties from one or more source objects to a target object.
Syntax
Object.assign(target, source1, source2, ...);
Example
let target = { name: 'John' };
let source = { age: 30 };
Object.assign(target, source);
console.log(target); // Output: { name: 'John', age: 30 }
Object.keys()
The Object.keys()
method returns an array of a given object’s own enumerable property names.
Syntax
Object.keys(objectName);
Example
const person = {
name: 'Alice',
age: 30
};
console.log(Object.keys(person)); // Output: ['name', 'age']
Object.values()
The Object.values()
method returns an array of a given object’s own enumerable property values.
Syntax
Object.values(objectName);
Example
const person = {
name: 'Alice',
age: 30
};
console.log(Object.values(person)); // Output: ['Alice', 30]
Object.entries()
The Object.entries()
method returns an array of a given object’s own enumerable string-keyed property [key, value] pairs.
Syntax
Object.entries(objectName);
Example
const person = {
name: 'Alice',
age: 30
};
console.log(Object.entries(person)); // Output: [['name', 'Alice'], ['age', 30]]
Object.freeze() and Object.seal()
Object.freeze()
: Prevents the modification of existing property attributes and values. It also prevents the addition of new properties.Object.seal()
: Prevents the addition of new properties and marks all existing properties as non-configurable.
Syntax
Object.freeze(objectName);
Object.seal(objectName);
Example
const person = {
name: 'Alice'
};
Object.freeze(person);
person.name = 'Bob'; // This will not change the name property
console.log(person.name); // Output: Alice
Advanced Object Patterns
Factory Functions
Factory functions are functions that create and return objects. They offer a way to create multiple objects with similar properties.
Example
function createPerson(name, age) {
return {
name: name,
age: age,
greet() {
console.log(`Hello, my name is ${this.name}`);
}
};
}
let person1 = createPerson('Alice', 30);
let person2 = createPerson('Bob', 25);
person1.greet(); // Output: Hello, my name is Alice
person2.greet(); // Output: Hello, my name is Bob
Singleton Pattern
The Singleton pattern ensures that a class has only one instance and provides a global point of access to it.
Example
const Singleton = (function() {
let instance;
function createInstance() {
return { value: 'Singleton Instance' };
}
return {
getInstance: function() {
if (!instance) {
instance = createInstance();
}
return instance;
}
};
})();
let instance1 = Singleton.getInstance();
let instance2 = Singleton.getInstance();
console.log(instance1 === instance2); // Output: true
Module Pattern
The Module pattern provides a way to create self-contained modules with private and public members.
Example
const CounterModule = (function() {
let count = 0;
return {
increment: function() {
count++;
console.log(count);
},
decrement: function() {
count--;
console.log(count);
}
};
})();
CounterModule.increment(); // Output: 1
CounterModule.increment(); // Output: 2
CounterModule.decrement(); // Output: 1
In this example, count
is private to the module, and increment
and decrement
are public methods.
Common Pitfalls and Debugging Tips
- Incorrect
this
Context: Ensure you understand howthis
works, especially in callbacks and methods. Use arrow functions or.bind()
when necessary. - Prototype Confusion: Be aware of the prototype chain and how inheritance works. Debug with
Object.getPrototypeOf()
andObject.prototype
methods. - Property Overwriting: Avoid unintentional property overwriting by carefully managing object properties, especially when merging objects.
Best Practices
- Use Descriptive Property Names: Choose clear and descriptive names for object properties to improve code readability.
- Encapsulate Data: Use objects to group related data and functions together. Consider using classes or factory functions for more complex scenarios.
- Leverage ES6 Features: Take advantage of ES6 features like classes, destructuring, and shorthand property names to write cleaner and more efficient code.
- Avoid Modifying Prototypes: Modifying native prototypes can lead to unexpected behavior. Prefer using object-specific methods and utilities.
Conclusion
Objects are a fundamental part of JavaScript and provide a powerful way to manage and manipulate data. By understanding how to create, access, and modify objects, as well as using advanced patterns and utilities, you can write more effective and maintainable code.
From basic object literals to advanced patterns like the Singleton and Module patterns, mastering objects will significantly enhance your JavaScript programming skills. Continue to explore and experiment with objects to deepen your understanding and improve your development practice.