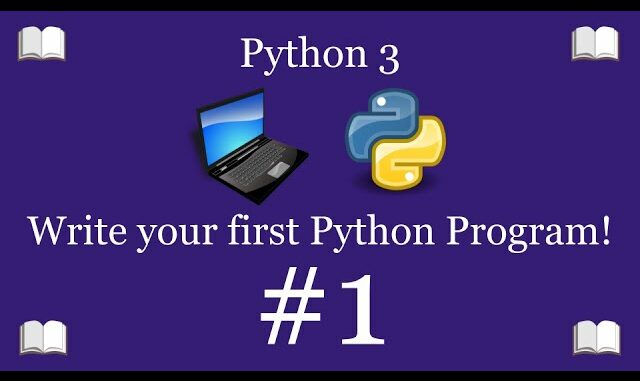
Introduction
Python is a versatile and powerful programming language that is widely used for a variety of applications, from web development to data science, machine learning, and more. Its simplicity and readability make it an excellent choice for beginners. In this comprehensive guide, we will walk you through the process of writing your first Python program. We will cover everything from setting up your development environment to writing and running your code. By the end of this guide, you will have a solid understanding of the basics of Python programming and be ready to tackle more complex projects.
Table of Contents
- Setting Up Your Development Environment
- Installing Python
- Installing an Integrated Development Environment (IDE)
- Writing Your First Python Program
- Understanding the Basics
- Writing Your Code
- Running Your Program
- Exploring Python Syntax and Concepts
- Variables and Data Types
- Basic Input and Output
- Conditional Statements
- Loops
- Functions
- Debugging and Troubleshooting
- Common Errors
- Debugging Techniques
- Next Steps and Resources
- Further Learning
- Building Your Own Projects
- Conclusion
Setting Up Your Development Environment
Before you can write and run Python code, you need to set up your development environment. This involves installing Python and choosing an Integrated Development Environment (IDE) to write your code.
Installing Python
Python is available for all major operating systems, including Windows, macOS, and Linux. Follow the steps below to install Python on your system:
- Download Python: Go to the official Python website at python.org.
- Select the Version: Click on the “Downloads” link in the top navigation bar and select the version of Python that is recommended for your operating system. For most users, the latest version is recommended.
- Run the Installer: Once the download is complete, open the installer file. Follow the prompts to install Python. Make sure to check the box that says “Add Python to PATH” before clicking the Install button. This will make it easier to run Python from the command line.
Installing an Integrated Development Environment (IDE)
An IDE is a software application that provides comprehensive facilities to computer programmers for software development. There are several IDEs available for Python, each with its own features and benefits. Some popular options include:
- PyCharm: A powerful and feature-rich IDE developed by JetBrains. It offers advanced code editing, debugging, and testing tools. You can download it from jetbrains.com/pycharm.
- Visual Studio Code: A lightweight and versatile code editor developed by Microsoft. It has excellent support for Python through extensions. You can download it from code.visualstudio.com.
- IDLE: The default IDE that comes with Python. It is simple and easy to use, making it a good choice for beginners.
For this guide, we will use Visual Studio Code (VS Code) due to its simplicity and popularity.
- Download and Install VS Code: Go to code.visualstudio.com and download the installer for your operating system. Follow the prompts to install it.
- Install the Python Extension: Open VS Code and click on the Extensions icon in the sidebar (or press
Ctrl+Shift+X
). Search for “Python” and click the Install button for the Python extension provided by Microsoft.
Writing Your First Python Program
With your development environment set up, you are ready to write your first Python program. We will start with a simple program that prints “Hello, World!” to the screen.
Understanding the Basics
Before we dive into writing code, let’s briefly discuss some basic concepts in Python:
- Syntax: Python syntax refers to the set of rules that define how a Python program is written and interpreted.
- Indentation: Python uses indentation to define blocks of code. Proper indentation is crucial for the code to run correctly.
- Comments: Comments are used to explain code and are ignored by the Python interpreter. Single-line comments start with a
#
.
Writing Your Code
- Create a New File: Open VS Code and create a new file by clicking on the “File” menu and selecting “New File” (or press
Ctrl+N
). Save the file with a.py
extension, such ashello.py
. - Write Your Code: In the new file, type the following code:
python
# This is a simple Python program
print("Hello, World!")
- The
print
function is used to display output on the screen. - The text inside the parentheses and quotes (
"Hello, World!"
) is the string that will be printed.
- The
Running Your Program
- Open a Terminal: Open the integrated terminal in VS Code by clicking on the “Terminal” menu and selecting “New Terminal” (or press
Ctrl+
`). - Navigate to Your File: Use the
cd
command to navigate to the directory where you saved your Python file. For example:shcd path/to/your/directory
- Run Your Program: In the terminal, type the following command and press Enter:
sh
python hello.py
You should see the output “Hello, World!” printed in the terminal.
Exploring Python Syntax and Concepts
Now that you have written and run your first Python program, let’s explore some basic Python syntax and concepts in more detail.
Variables and Data Types
Variables are used to store data that can be used and manipulated in your program. Python has several built-in data types, including integers, floats, strings, and booleans.
- Defining Variables:
python
name = "Alice"
age = 30
height = 5.5
is_student = True
name
is a string variable.age
is an integer variable.height
is a float variable.is_student
is a boolean variable.
- Printing Variables:
python
print(name)
print(age)
print(height)
print(is_student)
- Type Checking:
python
print(type(name))
print(type(age))
print(type(height))
print(type(is_student))
Basic Input and Output
You can use the input
function to get user input and the print
function to display output.
- Getting User Input:
python
user_name = input("Enter your name: ")
user_age = input("Enter your age: ")
- Displaying Output:
python
print("Hello, " + user_name + "!")
print("You are " + user_age + " years old.")
Conditional Statements
Conditional statements allow you to execute different blocks of code based on certain conditions. The most common conditional statements in Python are if
, elif
, and else
.
- Using
if
,elif
, andelse
:pythonage = int(input("Enter your age: "))
if age < 18:
print("You are a minor.")
elif age >= 18 and age < 65:
print("You are an adult.")
else:
print("You are a senior.")
- Logical Operators:
and
: ReturnsTrue
if both conditions are true.or
: ReturnsTrue
if at least one condition is true.not
: ReturnsTrue
if the condition is false.
Loops
Loops are used to execute a block of code repeatedly. Python supports for
loops and while
loops.
- Using a
for
Loop:pythonfor i in range(5):
print("Iteration:", i)
- Using a
while
Loop:pythoncount = 0
while count < 5:
print("Count:", count)
count += 1
Functions
Functions are reusable blocks of code that perform a specific task. They help to make your code more modular and organized.
- Defining a Function:
python
def greet(name):
print("Hello, " + name + "!")
- Calling a Function:
python
greet("Alice")
greet("Bob")
- Returning Values:
python
def add(a, b):
return a + bresult = add(3, 5)
print("Result:", result)
Debugging and Troubleshooting
As you write more complex programs, you may encounter errors. Learning how to debug and troubleshoot your code is an essential skill for any programmer.
Common Errors
- Syntax Errors: Occur when the code does not follow the correct syntax.
python
print("Hello, World!"
- Runtime Errors: Occur during the execution of the program.
python
print(1 / 0)
- Logical Errors: Occur when the code runs without errors but produces incorrect results.
python
result = 2 + 2
print("Result:", result) # Incorrect logic
Debugging Techniques
- Print Statements: Use
print
statements to check the values of variables and the flow of the program.pythondef add(a, b):
print("a:", a)
print("b:", b)
return a + bresult = add(3, 5)
print("Result:", result)
- Using a Debugger: Most IDEs, including VS Code, have built-in debuggers that allow you to set breakpoints, step through code, and inspect variables.
- Reading Error Messages: Carefully read error messages to understand what went wrong and where the error occurred.
Next Steps and Resources
Now that you have written your first Python program and learned some basic concepts, here are some next steps and resources to help you continue your Python journey.
Further Learning
- Online Tutorials and Courses:
- Books:
- “Automate the Boring Stuff with Python” by Al Sweigart
- “Python Crash Course” by Eric Matthes
- “Learning Python” by Mark Lutz
Building Your Own Projects
- Simple Projects:
- Calculator
- To-Do List
- Number Guessing Game
- Intermediate Projects:
- Web Scraper
- Personal Blog
- Weather App
- Advanced Projects:
- Machine Learning Model
- Web Application with Flask or Django
- Data Visualization Dashboard
Conclusion
Writing your first Python program is an exciting milestone in your programming journey. By setting up your development environment, understanding basic syntax and concepts, and practicing with simple projects, you can build a strong foundation in Python. As you continue to learn and explore, you will discover the many powerful features and capabilities of Python that make it one of the most popular programming languages in the world. Keep experimenting, building, and learning, and you will soon be able to tackle more complex and rewarding projects. Happy coding!