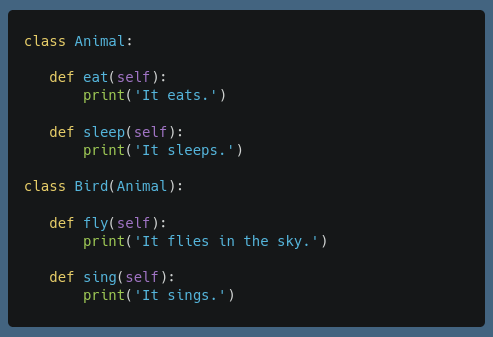
Understanding Inheritance
Inheritance is a fundamental concept in object-oriented programming (OOP) that allows you to create new classes (subclasses or derived classes) based on existing classes (base classes or parent classes). This promotes code reusability, hierarchy, and polymorphism.
Basic Inheritance
To create a subclass that inherits from a base class, use the following syntax:
class BaseClass:
# Base class attributes and methods
class Subclass(BaseClass):
# Subclass attributes and methods
The subclass inherits all attributes and methods of the base class. You can then add new attributes or methods to the subclass, or override existing ones.
Accessing Base Class Members
You can access members of the base class using the super()
function or directly using the base class name.
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
print("Generic animal sound")
class Dog(Animal):
def __init__(self, name, breed):
super().__init__(name)
self.breed = breed
def speak(self):
print(f"{self.name} barks!")
Method Overriding
You can override methods in the subclass to provide a different implementation.
class Animal:
def speak(self):
print("Generic animal sound")
class Dog(Animal):
def speak(self):
print("Woof!")
Method Overloading
Python doesn’t support method overloading in the same way as languages like C++ or Java. However, you can achieve similar behavior using default arguments or variable-length argument lists.
Multiple Inheritance
Python supports multiple inheritance, meaning a class can inherit from multiple base classes.
class Mammal:
def walk(self):
print("Walking")
class Aquatic:
def swim(self):
print("Swimming")
class Amphibian(Mammal, Aquatic):
pass
While multiple inheritance is powerful, it can lead to the “diamond problem” (multiple inheritance ambiguity). Use it cautiously.
The mro
Method
The mro()
method returns the method resolution order (MRO), which is the order in which Python searches for methods in a class hierarchy. This is important for understanding how method resolution works in multiple inheritance.
print(Amphibian.mro())
Abstract Base Classes (ABCs)
Abstract base classes define a common interface for derived classes. They cannot be instantiated themselves.
from abc import ABC, abstractmethod
class Shape(ABC):
@abstractmethod
def area(self):
pass
class Rectangle(Shape):
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
return self.width * self.height
Polymorphism
Polymorphism allows objects of different types to be treated as if they were of the same type. This is enabled by inheritance.
def make_sound(animal):
animal.speak()
class Dog:
def speak(self):
print("Woof!")
class Cat:
def speak(self):
print("Meow!")
dog = Dog()
cat = Cat()
make_sound(dog) # Output: Woof!
make_sound(cat) # Output: Meow!
Best Practices for Inheritance
- Use inheritance judiciously. It should represent an “is-a” relationship.
- Favor composition over inheritance when possible.
- Keep inheritance hierarchies shallow.
- Use abstract base classes to define interfaces.
- Test your code thoroughly.
Advanced Inheritance Topics
- Mixins: Classes that provide additional functionality to other classes without representing an “is-a” relationship.
- Diamond problem: Understand and mitigate the issues associated with multiple inheritance.
- Metaclasses: Create classes that create other classes.
Conclusion
Inheritance is a powerful tool for code organization and reusability in Python. By understanding its concepts and best practices, you can effectively design and implement class hierarchies. However, use inheritance wisely and consider alternative approaches like composition when appropriate.