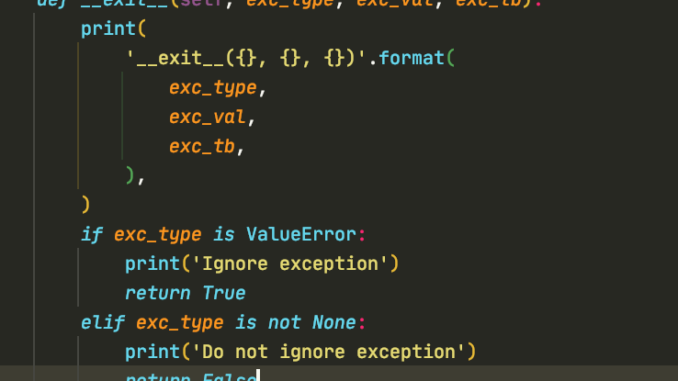
Understanding Context Managers
A context manager is a Python object that defines a runtime context for a block of code. It ensures that specific actions are performed at the beginning and end of the code block, regardless of how the block terminates (normal execution, exceptions, or other causes). This is often referred to as the “with” statement pattern.
The with
Statement
The with
statement is the primary mechanism for using context managers in Python. It provides a clean and reliable way to manage resources that need to be acquired and released in a specific order.
with context_manager() as resource:
# Code that uses the resource
The with
statement guarantees that the __exit__
method of the context manager will be called, even if an exception occurs within the block.
Creating Context Managers
There are two primary ways to create context managers:
1. Using the @contextlib.contextmanager
Decorator
This method is suitable for simple context managers that don’t require complex setup or teardown logic.
from contextlib import contextmanager
@contextmanager
def my_context_manager():
# Setup code
try:
yield # Return a value to the with statement
except Exception as e:
# Handle exceptions
finally:
# Cleanup code
2. Implementing the __enter__
and __exit__
Methods
For more complex context managers, you can define a class that implements the __enter__
and __exit__
methods.
class MyContextManager:
def __enter__(self):
# Setup code
return self # Return a value to the with statement
def __exit__(self, exc_type, exc_value, traceback):
# Cleanup code
if exc_type is not None:
# Handle exceptions
Common Use Cases for Context Managers
- File Handling:
Python
with open('file.txt', 'r') as f: data = f.read()
- Database Connections:
Python
import sqlite3 with sqlite3.connect('mydatabase.db') as conn: cursor = conn.cursor() # Perform database operations
- Locking:
Python
import threading lock = threading.Lock() with lock: # Critical section
- Resource Management:
Python
import resource with resource.open('resource_name') as resource: # Use the resource
Custom Context Managers
You can create custom context managers for specific use cases. For example:
-
Timing a code block:
Pythonimport time @contextmanager def timer(): start_time = time.time() yield end_time = time.time() print(f"Elapsed time: {end_time - start_time:.2f} seconds")
-
Managing temporary files:
Pythonimport tempfile @contextmanager def temporary_file(suffix='.tmp'): temp_file = tempfile.NamedTemporaryFile(suffix=suffix, delete=False) try: yield temp_file.name finally: temp_file.close()
Advanced Topics
- Nested Context Managers: Use multiple
with
statements to manage nested resources. - Asynchronous Context Managers: Use
async with
for asynchronous operations. - Contextlib Modules: Explore additional context manager utilities in the
contextlib
module.
Conclusion
Context managers are a powerful tool for managing resources and ensuring proper cleanup in Python. By understanding their core concepts and common use cases, you can write more robust and efficient code.