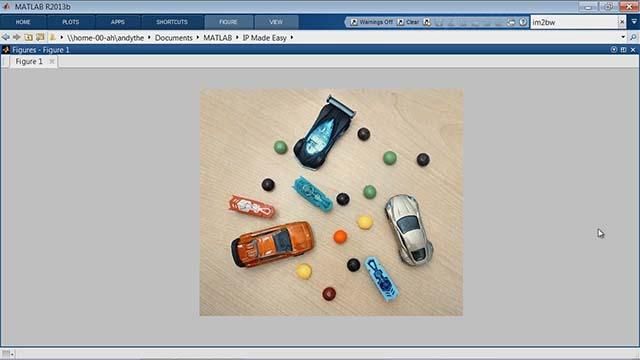
Image processing and computer vision are indispensable fields in modern technology, with applications ranging from medical imaging and surveillance to autonomous vehicles and augmented reality. MATLAB, with its extensive set of built-in functions, toolboxes, and libraries, offers a comprehensive platform for performing image processing and computer vision tasks. In this comprehensive article, we will explore various techniques, methods, and best practices for performing image processing and computer vision tasks in MATLAB.
Table of Contents
- Introduction to Image Processing and Computer Vision
- Basics of Image Representation
- Loading and Displaying Images
- Image Enhancement and Filtering
- Image Segmentation
- Feature Extraction and Object Detection
- Image Registration and Alignment
- Geometric Transformations
- Morphological Operations
- Image Restoration and Deblurring
- Deep Learning for Computer Vision
- Practical Applications
- Best Practices and Tips
- Conclusion
1. Introduction to Image Processing and Computer Vision
Image processing involves manipulating and analyzing images to extract useful information or enhance specific features. Computer vision, on the other hand, focuses on the interpretation of images to understand the content and context of visual data. Both fields play a crucial role in various applications, including medical imaging, surveillance, robotics, and more.
2. Basics of Image Representation
Images are typically represented as matrices or arrays of pixel values, where each pixel corresponds to a specific location in the image and contains intensity or color information. In MATLAB, grayscale images are represented as 2D matrices, while color images are represented as 3D matrices with separate channels for each color component (e.g., red, green, blue).
3. Loading and Displaying Images
MATLAB provides functions for loading and displaying images from various file formats, including JPEG, PNG, TIFF, and more.
% Load and display an image
img = imread('image.jpg');
imshow(img);
title('Original Image');
4. Image Enhancement and Filtering
Image enhancement techniques aim to improve the visual quality of images by adjusting brightness, contrast, and sharpness. MATLAB provides functions for performing various image enhancement operations, such as histogram equalization, contrast stretching, and noise reduction.
% Enhance image contrast using histogram equalization
img_eq = histeq(img);
% Display the enhanced image
imshow(img_eq);
title('Enhanced Image (Histogram Equalization)');
5. Image Segmentation
Image segmentation involves partitioning an image into multiple regions or segments to simplify analysis and facilitate object detection. MATLAB offers functions for performing image segmentation using techniques such as thresholding, region growing, and clustering.
% Perform image segmentation using Otsu's method
threshold = graythresh(img);
bw = imbinarize(img, threshold);
% Display the segmented image
imshow(bw);
title('Segmented Image (Otsu)');
6. Feature Extraction and Object Detection
Feature extraction involves identifying and extracting meaningful information or features from images, such as edges, corners, and keypoints. MATLAB provides functions for feature extraction and object detection using techniques like Harris corner detection, SURF (Speeded-Up Robust Features), and HOG (Histogram of Oriented Gradients).
% Detect corners using Harris corner detector
corners = detectHarrisFeatures(img);
% Display the detected corners
imshow(img);
hold on;
plot(corners);
title('Detected Corners (Harris)');
7. Image Registration and Alignment
Image registration involves aligning multiple images to a common coordinate system to facilitate comparison or fusion. MATLAB provides functions for image registration using techniques such as affine transformation, intensity-based registration, and feature-based registration.
% Register two images using feature-based registration
moving_img = imread('moving_image.jpg');
fixed_img = imread('fixed_image.jpg');
registered_img = imregister(moving_img, fixed_img, 'rigid');
% Display the registered image
imshowpair(fixed_img, registered_img, 'montage');
title('Registered Images');
8. Geometric Transformations
Geometric transformations involve changing the spatial orientation, scale, or perspective of images. MATLAB provides functions for performing geometric transformations, including translation, rotation, scaling, and affine transformations.
% Perform image rotation
theta = 30; % Rotation angle (degrees)
rotated_img = imrotate(img, theta);
% Display the rotated image
imshow(rotated_img);
title('Rotated Image');
9. Morphological Operations
Morphological operations are used to analyze and manipulate the shape and structure of objects in images. MATLAB offers functions for performing morphological operations such as dilation, erosion, opening, and closing.
% Perform morphological dilation
se = strel('disk', 5); % Structuring element
dilated_img = imdilate(img, se);
% Display the dilated image
imshow(dilated_img);
title('Dilated Image');
10. Image Restoration and Deblurring
Image restoration techniques aim to remove noise and artifacts from images to restore their original appearance. MATLAB provides functions for image restoration and deblurring using techniques such as Wiener filtering, blind deconvolution, and total variation regularization.
% Restore blurred image using Wiener filtering
blurred_img = imread('blurred_image.jpg');
estimated_noise = 0.1 * var(double(blurred_img(:))); % Estimated noise variance
restored_img = deconvwnr(blurred_img, psf, estimated_noise);
% Display the restored image
imshow(restored_img);
title('Restored Image (Wiener Filtering)');
11. Deep Learning for Computer Vision
Deep learning techniques, particularly convolutional neural networks (CNNs), have revolutionized computer vision tasks such as image classification, object detection, and semantic segmentation. MATLAB provides tools and frameworks for training, fine-tuning, and deploying deep learning models for various computer vision applications.
% Train a CNN for image classification
net = alexnet; % Pre-trained CNN
imds = imageDatastore('data', 'LabelSource', 'foldernames');
options = trainingOptions('sgdm', 'MaxEpochs', 10);
trained_net = trainNetwork(imds, net, options);
12. Practical Applications
Image processing and computer vision have diverse applications across various domains:
- Medical Imaging: Diagnosing diseases, analyzing medical scans (e.g., MRI, CT), and surgical navigation.
- Surveillance and Security: Monitoring and analyzing video feeds for security and surveillance purposes.
- Autonomous Vehicles: Object detection, lane detection, and scene understanding for autonomous driving systems.
- Remote Sensing: Analyzing satellite images for environmental monitoring, agriculture, and urban planning.
- Augmented Reality: Overlaying digital information on real-world scenes for gaming, navigation, and industrial applications.
13. Best Practices and Tips
- Preprocess Images: Clean and preprocess images before performing analysis to improve the accuracy of results.
- Choose Appropriate Techniques: Select the right image processing and computer vision techniques based on the specific requirements and characteristics of the images.
- Validate and Evaluate: Validate the performance of algorithms using appropriate metrics and evaluation techniques.
- Optimize Performance: Optimize the implementation of algorithms for efficiency and computational performance, especially for real-time applications.
- Consider Robustness: Ensure that image processing and computer vision algorithms are robust to variations in lighting conditions, noise levels, and image quality.
- Keep Learning: Stay updated with the latest developments and advancements in image processing and computer vision by exploring research papers, attending conferences, and participating in online courses.
- Collaborate and Share: Collaborate with peers and experts in the field, and share your knowledge and experiences to foster innovation and growth in the community.
14. Conclusion
Image processing and computer vision are essential fields that play a critical role in various applications, from healthcare and automotive to security and entertainment. MATLAB provides a powerful platform for performing a wide range of image processing and computer vision tasks, thanks to its extensive set of functions, toolboxes, and libraries. By leveraging MATLAB’s capabilities and following best practices, researchers, engineers, and developers can efficiently analyze images, extract valuable information, and build robust computer vision systems to address real-world challenges and drive innovation in diverse domains. Whether you are a beginner exploring the basics of image processing or an experienced practitioner working on advanced computer vision projects, MATLAB offers the tools and resources you need to succeed in your endeavors.